关键词:
Swoole+Redis+webSocket实现点对点即时聊天
场景
Swoole+Redis+webSocket实现点对点即时聊天。
webSocket服务端代码
我们需要通过Laravel Command来实现,因为Swoole只能运行在PHP CLI模式下。
1.生成Command类
php artisan make:command SwooleServer
2.编写webSocket Server逻辑
<?php
namespace App\\Console\\Commands;
use Illuminate\\Console\\Command;
use Illuminate\\Support\\Facades\\Redis;
class SwooleServer extends Command
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'swoole:server';
/**
* The console command description.
*
* @var string
*/
protected $description = 'swoole websocket';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
parent::__construct();
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
$server = new \\Swoole\\WebSocket\\Server("0.0.0.0", 9502);
$server->on('open', function($server, $req)
echo "connection open: $req->fd\\n";
);
$server->on('message', function($server, $frame)
echo "received message: $frame->data\\n";
$receive_data=json_decode($frame->data);
$redis=new \\Redis();
$redis->connect('127.0.0.1', 6379);
$redis->auth('123456');
if($receive_data->type=='bind')
//关系绑定
$redis->set('fd_'.$receive_data->name,$frame->fd);
$redis->set('name_'.$frame->fd,$receive_data->name);
else
//消息发送
$data['fd']=$frame->fd;
$data['name']=$receive_data->name;
$data['data']=$receive_data->msg;
$server->push($redis->get('fd_'.$receive_data->touser), json_encode($data));
$server->push($frame->fd, json_encode($data));
);
$server->on('close', function($server, $fd)
echo "connection close: $fd\\n";
//取消关系绑定
$redis=new \\Redis();
$redis->connect('127.0.0.1', 6379);
$redis->auth('123456');
$redis->del('fd_'.$redis->get('name_'.$fd));
$redis->del('name_'.$fd);
);
$server->on('Shutdown', function($server)
//kill -15 PID 才会触发
echo "Shutdown。。。\\n";
$redis=new \\Redis();
$redis->connect('127.0.0.1', 6379);
$redis->auth('123456');
//取消所有关系绑定
foreach($server->connections as $fd)
$redis->del('fd_'.$redis->get('name_'.$fd));
$redis->del('name_'.$fd);
);
$server->start();
前端代码
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>聊天室</title>
<script src="http://libs.baidu.com/jquery/2.0.0/jquery.min.js"></script>
</head>
<style>
ul,lilist-style: none;padding: 0;margin: 0;
bodywidth: 50%;float: left;margin: 5px 25%;
.msg_boxwidth: 100%;border:1px solid #ddd;height: 500px; float: left;overflow: scroll;
.msg_box lipadding: 10px;border-bottom:1px solid #ddd;
.op_boxwidth: 100%;float: left;
.op_box textareawidth: 96%;float: left;padding: 2%;border:1px solid #ddd;height: 50px;resize: none;
.op_box awidth: 200px;display: block;float: right;height: 30px;line-height: 30px;background: #ddd;color: black;text-align: center;text-underline-style: none;margin-top: 5px;
</style>
<body>
<ul class="msg_box"></ul>
<div class="op_box">
<textarea name="msg" placeholder="请输入消息"></textarea>
<a href="javascript:;" class="send_btn">发送</a>
</div>
</body>
<script>
var data='';
var name = window.prompt('请输入昵称', 'jungshen');
var touser=window.prompt('请输入好友昵称', 'mirror');
$('title').html('您是:'+name+'正在与'+touser+'聊天_'+$('title').html());
if(name)
var ws = new WebSocket("ws://172.20.31.192:9502");
ws.onopen = function(evt)
console.log("Connection open ...");
//ws.send("Hello WebSockets!");
data='name':name,'type':'bind'
ws.send(JSON.stringify( data ));
;
ws.onmessage = function(evt)
console.log( "Received Message: " + evt.data);
dataObj=eval('('+evt.data+')');
$('.msg_box').append('<li>'+dataObj.name+':'+dataObj.data+'</li>')
//ws.close();
;
ws.onclose = function(evt)
console.log("Connection closed.");
;
ws.onerror = function(evt)
console.log('error');
;
$('.send_btn').click(function()
var msg=$('textarea').val();
if(msg)
data='name':name,'msg':msg,'type':'msg','touser':touser
ws.send(JSON.stringify( data ));
$('textarea').val('');
);
document.onkeydown=function(event)
if(event.keyCode==13)
$('.send_btn').click();
return false;
</script>
</html>
```
# 预览
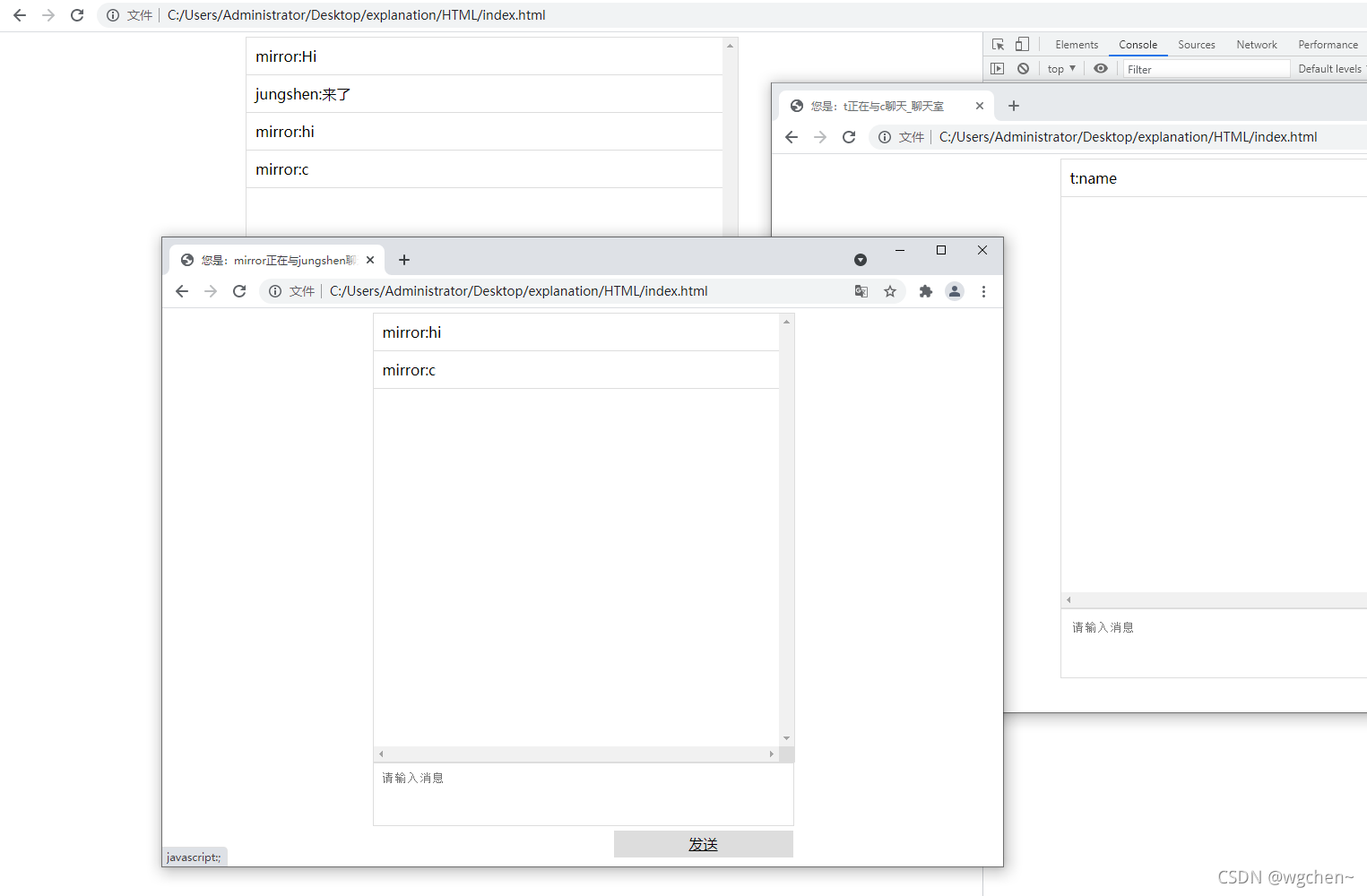
基于swoole搭建websocket服务详解
...节将会详解以下 4 个问题:什么是swoole?什么是Websocket?如何基于Swoole构建WebSocket服务?基于Swoole的WebSocket服务和Http服务是什么关系?一、Swoole 简介Swoole是一个面向生产环境的PHP异步网络通信引擎,使PHP开发人员能... 查看详情
swoole入门-websocket服务
参考技术AWebSocket协议是基于TCP的一种新的网络协议。他实现了浏览器与服务器全双工通信—允许服务器主动发送信息给客户端。建立在TCP协议之上性能开销小性能高效客户端可以与任意服务器通信协议标识符号wswss持久化网络通... 查看详情
消息服务器websocket高并发分布式swoole架构思路
参考技术A消息服务器使用socket,为避免服务器过载,单台只允许500个socket连接,当一台不够的时候,扩充消息服务器是必然,问题来了,如何让链接在不同消息服务器上的用户可以实现消息发送呢?要实现消息互通就必须要让... 查看详情
swoole弹幕系统
...以及清空缓存 二、弹幕服务端1、danmu-server使用swoole_websocket_server,中间是子进程,最下面是redis服务器2、web-server是网页http请求,可以产生的动态推送 查看详情
php如何实现websocket
php有可用的websocket库,不需要php-fpm。目前比较成熟的有swoole(swoole.com),和workman(workman.net)swoole是c写的php扩展,效率比nodejs还要高,workman是纯php实现,两者都号称可以实现并发百万TCP连接。给你个例子:这个要通过cmd运行... 查看详情
laravel+swoole打造im简易聊天室(代码片段)
...用场景:实现简单的即时消息聊天室(一)扩展安装(二)webSocket服务端代码(三)客户端实现应用场景:实现简单的即时消息聊天室(一)扩展安装peclinstallswoole安装完成后可以通过以下命令检测Swoole是否安装成功php-m|grepswoole(二)w... 查看详情
laravel+swoole打造im简易聊天室(代码片段)
...用场景:实现简单的即时消息聊天室(一)扩展安装(二)webSocket服务端代码(三)客户端实现应用场景:实现简单的即时消息聊天室(一)扩展安装peclinstallswoole安装完成后可以通过以下命令检测Swoole是否安装成功php-m|grepswoole(二)w... 查看详情
swoole和workerman哪个更易开发
...加密、http2.0、异步mysql驱动、异步redis驱动、异步的http/websocket客户端、process、lock、atomic、table。另外Swoole2.0内置了PHP原生协程的支持,PHP代码也可以使用类似于Go语言的协程来实现高并发的网络服务器。外部依赖上workerman需要... 查看详情
swoole-websocket毫秒定时器(代码片段)
Swoole毫秒定时器简介别名应用Ws.phpindex.html简介常规定时器:linuxcrontabSwoole定时器swoole_timer_tickswoole_timer_after别名tick()、after()、clear()都拥有一个函数风格的别名类静态方法函数风格别名Swoole\\Timer::tick()swoole_timer_tick()Swoole\\T 查看详情
swoole-websocket毫秒定时器(代码片段)
Swoole毫秒定时器简介别名应用Ws.phpindex.html简介常规定时器:linuxcrontabSwoole定时器swoole_timer_tickswoole_timer_after别名tick()、after()、clear()都拥有一个函数风格的别名类静态方法函数风格别名Swoole\\Timer::tick()swoole_timer_tick()Swoole\\T 查看详情
学习swoole的心得
...秒定时器,异步文件读写,异步DNS查询。Swoole内置了Http/WebSocket服务器端/客户端、Http2.0服务器端。Swo 查看详情
github上都有哪些比较有趣的php项目?
...框架。php-webim,基于swoole实现的Web即时聊天工具具,支持websocket+httpcomet长链接推送,可以发送文字内容和图片。使用PHP代码实现异步框架。如果说swoole是node.js的升级版,react.php就是标准的PHP版本node.jsworkerman,类似swoole,不过是... 查看详情
swoole如何处理高并发以及异步i/o的实现
...定时器,异步文件读写,异步DNS查询。Swoole还内置了Http/WebSocket服务器端/客户端、Http2.0服务器端。2.Swoole可以广泛应用于互联网、移动通信、企业软件、网络游戏、物联网、车联网、智能家庭等领域。使用PHP+Swoole作为网络通信... 查看详情
php只能做网站?基于swoole+websocket开发双向通信应用(代码片段)
前言众所周知,PHP用于开发基于HTTP协议的网站应用非常便捷。而HTTP协议是一种单向的通信协议,只能接收客户端的请求,然后响应请求,不能主动向客户端推送信息。因此,一些实时性要求比较高的应用,如实时聊天、直播应... 查看详情
swoole学习:初识swoole(代码片段)
...模块。可以方便快速的实现 TCP/UDP服务、高性能Web、WebSocket服务、物联网、实时通讯、游戏、微服务等,使 PHP 不再局限于传统的Web领域。在我的理解里,swoole就是一个PHP的拓展,类似于php_mbstring.dll、php_redis.dll等拓展... 查看详情
swoole系列2.4websocket服务
WebSocket服务对于Web应用来说,最主流的当然就是我们之前学习过的Http、TCP、UDP这一类的应用。但是,最近这些年,特别是HTML5成为主流之后,WebSocket应用日益丰富起来。要知道,之前我们在做后台时,如果... 查看详情
使用php+swoole实现的网页即时聊天工具:phpwebim
...步非阻塞Server,可以同时支持数百万TCP连接在线同时支持websocket+comet2种兼容协议,可用于所有种类的浏览器包括IE拥有完整的UI界面支持单聊/群聊/组聊等功能支持发送表情支持永久保存聊天记录基于ServerPUSH的即时内容更新,登... 查看详情
基于redis发布订阅和websocket实现聊天室功能
参考技术A同时在做消息的持久化的时候,可以利用Redis的Zset的特性来对历史消息进行存储。 查看详情